This is how to achieve equal spacing between grid elements and RecyclerView
borders. Applicable for both GridLayoutManager
and StaggeredGridLayoutManager
. No modification of existing Adapter
is required.
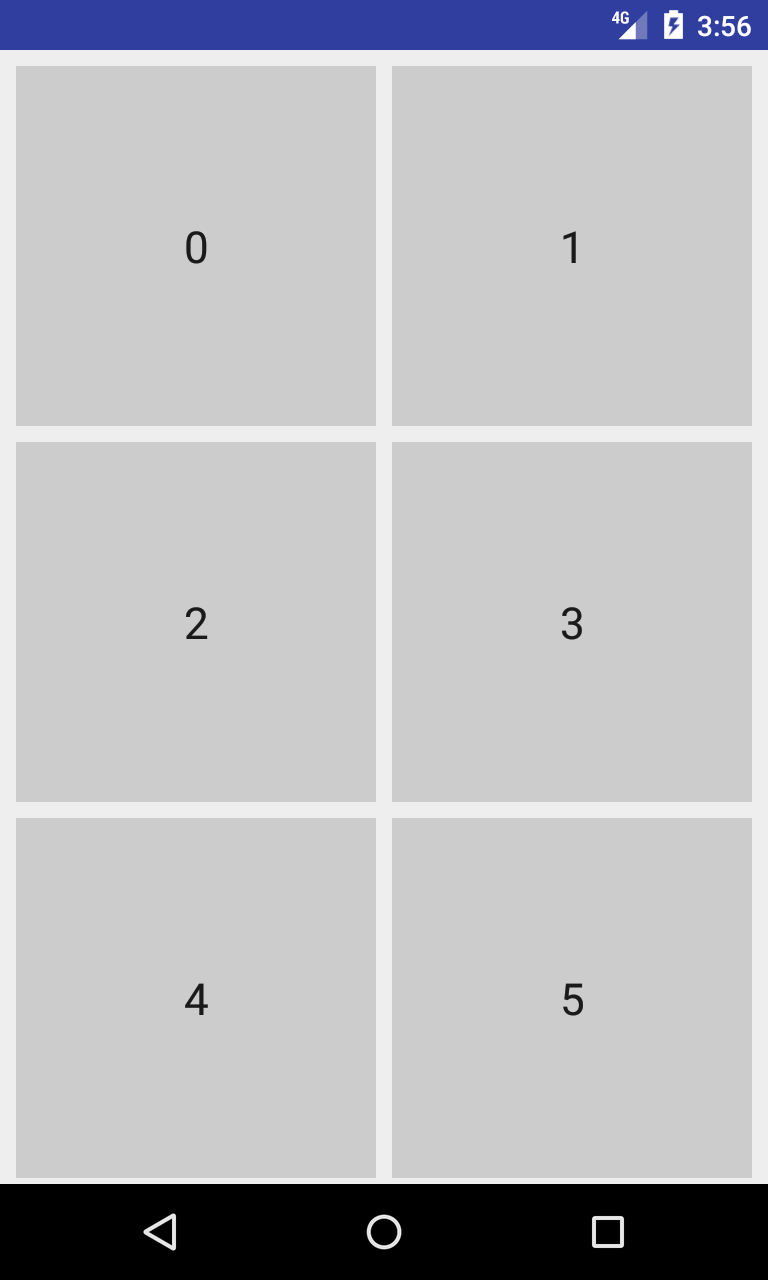
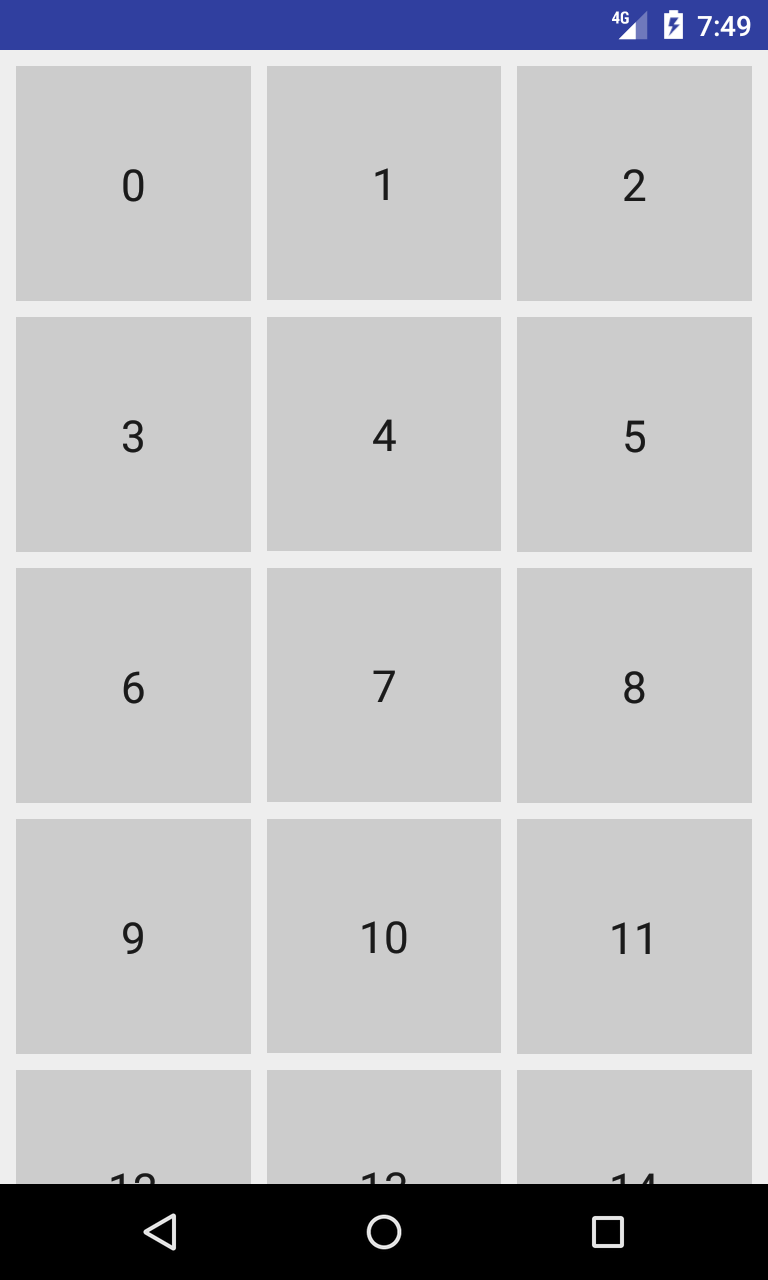
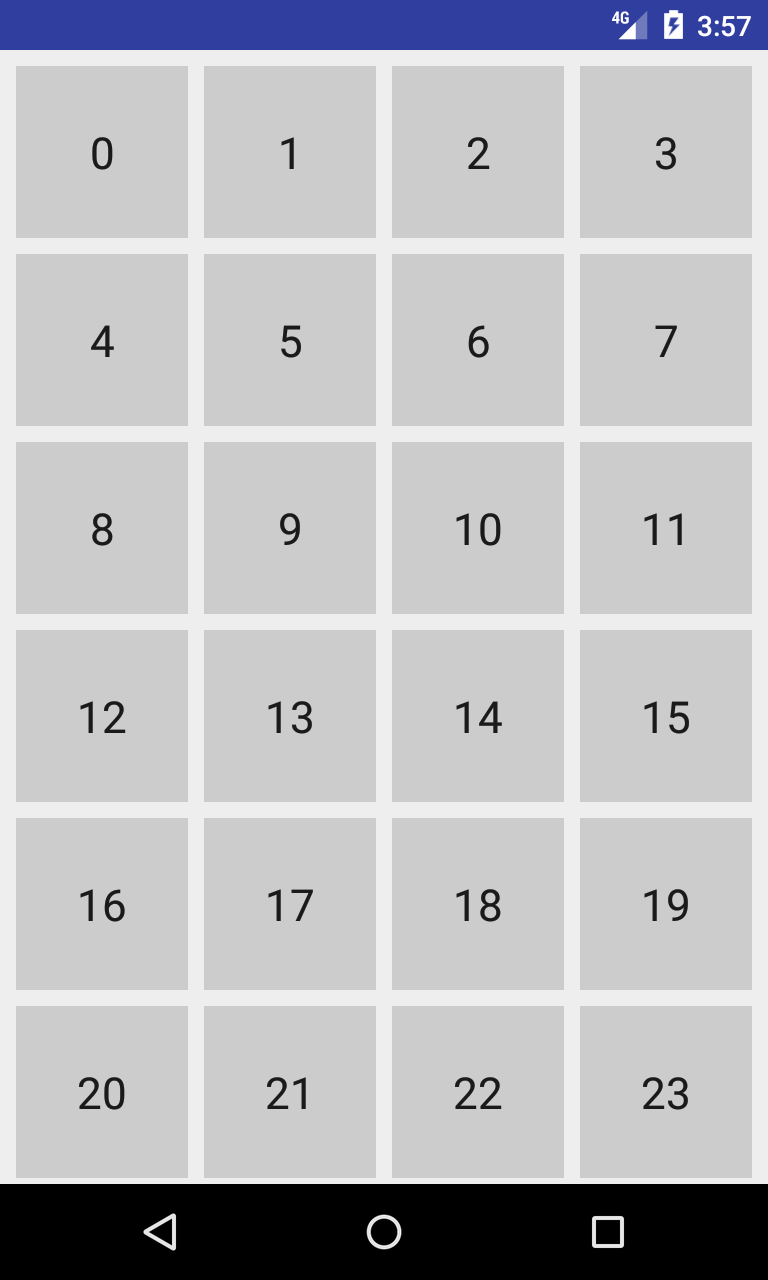
The simple trick is to combine RecyclerView.ItemDecoration
and RecyclerView
paddings:
// initialise RecyclerView
final RecyclerView recyclerView = findViewById(R.id.recycler_view);
final GridLayoutManager layoutManager = new GridLayoutManager(this, spanCount);
recyclerView.setLayoutManager(layoutManager);
recyclerView.setAdapter(new Adapter(this));
final int spacing = getResources().getDimensionPixelSize(R.dimen.recycler_spacing) / 2;
// apply spacing
recyclerView.setPadding(spacing, spacing, spacing, spacing);
recyclerView.setClipToPadding(false);
recyclerView.setClipChildren(false);
recyclerView.addItemDecoration(new RecyclerView.ItemDecoration() {
@Override
public void getItemOffsets(Rect outRect, View view, RecyclerView parent, RecyclerView.State state) {
outRect.set(spacing, spacing, spacing, spacing);
}
});
In the snippet above we are dividing desired spacing by two. We need this because we are applying half of the spacing to each element and RecyclerView
borders. This way all items will be surrounded by equal spacing.